I just solved a problem I was having in a Java Swing application getting input focus on a JDialog in a homemade text editor I use on my Mac OS X systems. Using my initial Java code the JDialog was properly getting input focus the first time it was displayed, but it was not properly getting input focus when it was displayed a second (or any subsequent) time. Since I like to write software that I also enjoy using, I really wanted to solve this perplexing Swing problem.
Fortunately, after some digging around and experimenting, I solved this problem. I'll spare you all the details of everything I tried, and just share with you the Java source code that ended up being the solution to my Swing/JDialog problem.
The JDialog input focus source code
Here's the "JDialog input focus" source code for my showDialog()
method from one of my controller classes in my Swing application:
public void showDialog() { String selectedText = mainFrame.getWikiEditingArea().getSelectedText(); if (selectedText != null) { hyperlinkDialog.getDescriptionTextField().setText(selectedText); } else { hyperlinkDialog.getDescriptionTextField().setText(""); } hyperlinkDialog.getUrlTextField().setText(""); hyperlinkDialog.getTitleTextTextField().setText(""); hyperlinkDialog.setLocationRelativeTo(mainFrame); // this solves the problem where the dialog was not getting // focus the second time it was displayed SwingUtilities.invokeLater(new Runnable() { public void run() { hyperlinkDialog.getUrlTextField().requestFocusInWindow(); } }); hyperlinkDialog.setModal(true); hyperlinkDialog.setVisible(true); }
The source code I've shown in bold above shows the "trick" I used to solve my JDialog input focus problem. The SwingUtilities invokeLater()
method was the key to my success. It's really important to get your Swing events on the Java Event Dispatch Thread (EDT), and the invokeLater method is usually the right way to do this.
What it looks like
For the record, here's what my application and this popup dialog looks like when it's displayed. Note that that when the dialog is displayed it has input focus, and specifically the first field in the dialog has input focus:
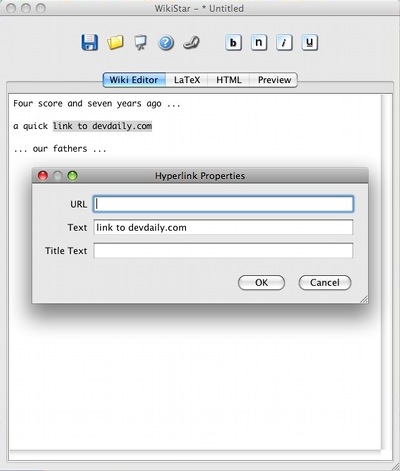
I know I tend to be a little particular about things :) but I sure am happier when I can just keep typing at the keyboard without having to click a dialog to give it input focus. With this application I've defined a keystroke that displays this dialog, and then I can also accept my input on the dialog with the [Enter] key, or discard the dialog by hitting the [Esc] key.
Version notes
This solution works for me using Java version 1.5.0_16 on Mac OS X version 10.5.x.