When I first moved to Alaska I took a really nice camera with me, and I took some fun photos I would have never thought to take with a regular camera. In this photo I was more or less laying down in a field of wildflowers (some weeds) at the Talkeetna Airport, bees and everything.
Scala, Java, Unix, MacOS tutorials (page 90)
These are some terrific words from a blog post titled, I’m Not The Radical Left, I’m The Humane Middle:
I believe in full LGBTQ rights.
I believe we should protect the planet.
I believe everyone deserves healthcare.
I believe all religions are equally valid.
I believe the world is bigger than America.
I believe to be “pro-life,” means to treasure all of it.
I believe whiteness isn’t superior and it is not the baseline of humanity.
I believe we are all one interdependent community.
I believe people and places are made better by diversity.
I believe people shouldn’t be forced to abide by anyone else’s religion.
I believe non-American human beings have as much value as American ones.
I believe generosity is greater than greed, compassion better than contempt, and kindness superior to derision.
I believe there is enough in this world for everyone: enough food, enough money, enough room, enough care — if we unleash our creativity and unclench our fists.
I just noticed that some of the MySQL files on this website had grown very large, so I wanted to be able to list all of the files in the MySQL data directory and sort them by filesize, with the largest files shown at the end of the listing. This ls
command did the trick, resulting in the output shown in the image:
ls -Slhr
The -S
option is the key, telling the ls
command to sort the file listing by size. The -h
option tells ls
to make the output human readable, and -r
tells it to reverse the output, so in this case the largest files are shown at the end of the output.
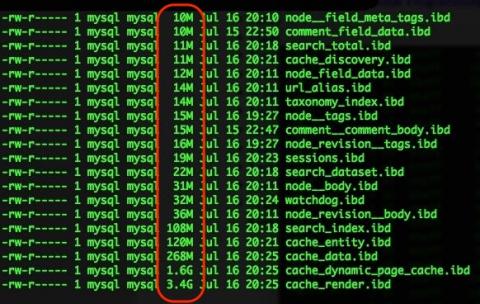
When I originally saw the first image, I thought it was a “This would be nice in Scala 3 (Dotty)” idea. But then I found the “No parentheses in control structures” pull request shown in the second image. Here’s a link to that pull request.
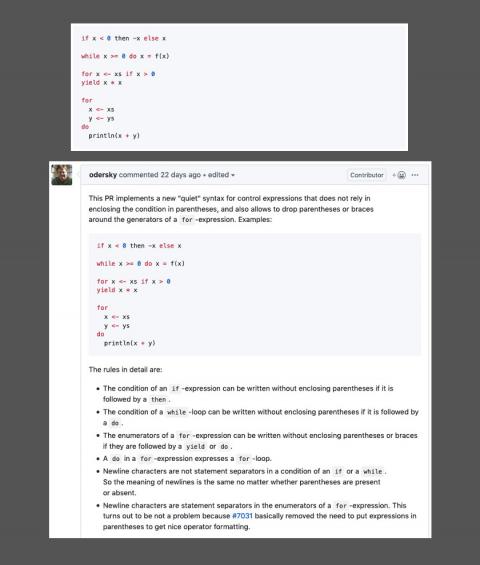
From a friend on Facebook, this is a great way to look at the two possibilities about climate change:
- If scientists are wrong, hey, we’ll have a cleaner world
- If they’re right, we’re all dead
Your choice.
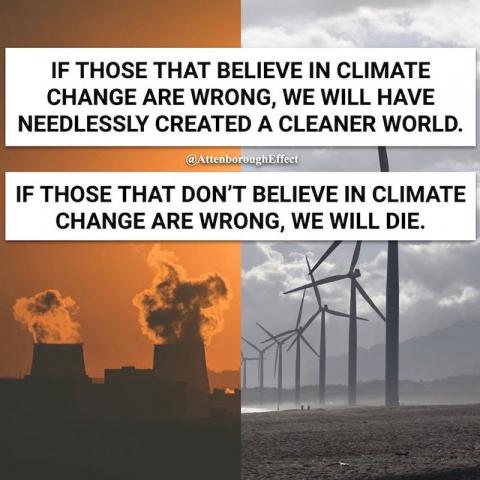
I’ve been working with Flutter and Dart for several weeks now, and I was surprised to read several times that Dart is single-threaded, knowing that it has a concept of a Future (or futures) and async methods. Last night I read this excellent article about Dart’s event loop, which sums up Dart futures very nicely in that statement:
“the code of these Futures will be run as soon as the Event Loop has some time. This will give the user the feeling that things are being processed in parallel (while we now know it is not the case).”
Earlier in the article the author also states:
“An async method is NOT executed in parallel but following the regular sequence of events, handled by the Event Loop, too.”
So, in summary, Dart has a single-threaded event loop, and futures and async methods aren’t handled by a separate thread; they’re handled by the single-threaded event loop whenever it has nothing else to do.
I just wanted to note this here for myself today, but for many more details, please see that article, which also discusses Dart isolates, which are like a more primitive form of Akka actors.
Today I learned that for several reasons, Flutter Debug mode may be significantly slower than Production mode. As just one reason, Debug mode is compiled using JIT while Production mode uses AOT. You can read more about the reasons on the Flutter UI Performance page.
A very important note on that page is that if you’re using a real hardware device (as opposed to an emulator), you can run your code in Profile mode like this:
If you ever need to run multiple Dart futures in parallel (simultaneously), I can confirm that this approach works:
So I pour some root beer in a Ball jar glass I use to drink from. I put some ice in it, then set it on the counter because I realize I left my glasses in the bedroom. In the bedroom I hear a cracking noise from the kitchen, but when I come back I can’t figure out what it was, so I assume it was the ice cracking in the root beer.
I pick up the Ball glass, take it to the computer area, then realize I now left my glasses in the kitchen. So I start walking to the kitchen, and the bottom of one of my feet feels a little wet. I can’t make sense of that, unless the Ball glass already started perspiring and dripped.
I come back to the computer area and find a little puddle of root beer under my glass. “Oh,” I think, “the root beer must have overflowed after I put the ice in. Strange I didn’t feel it on my hands.”
So I pick up the Ball glass and think, “Huh, that’s a lot of root beer under there,” so I take it back to the kitchen to rinse the glass under the sink. When I put the glass under the sink the bottom explodes and falls out.
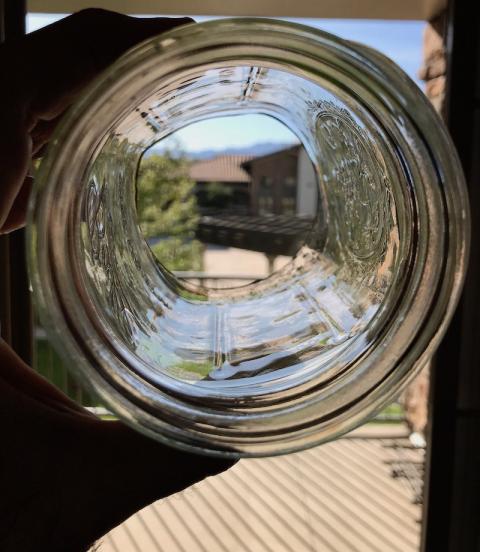
Over the last two days I started to get my Just Be notifications working on Android. I’m rewriting the app using Flutter, and this is what a notification currently looks like if you do a long-press on the app icon after receiving a notification.
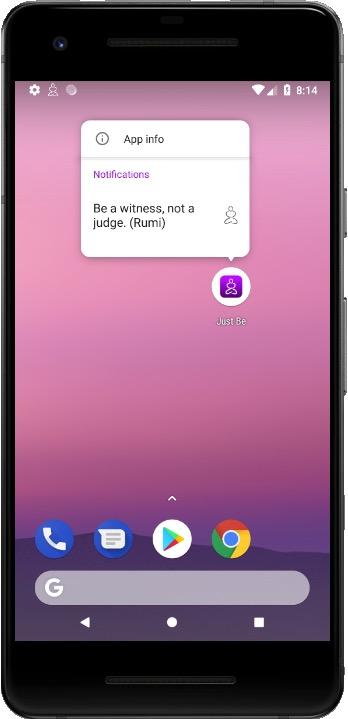
Notes from September 24, 2016:
Doctor: I’d like to collect a bone marrow sample ...
*Al runs out of the hospital in a hospital gown, screaming like a little girl*
(later, after they caught me)
Doctor: The next time you break out in a rash, hives, or blisters, I want you to have those biopsied.
Me: Is there going to be any part of our relationship that doesn’t involve a lot of pain on my part?
Doc: Yes, pee in this cup, and we’ll look at it under a fluorescent light to see if you have the same disease that King George III had.
Me: The crazy one?
Doc: Yes.
Me: Cool.
I recently created a command I named ffx
that lets you search your filesystem for files that contain multiple strings or regular expressions. This post describes and demonstrates its capabilities. (There’s a little video down below if you want to see how it works before reading about it.)
If you need to get a random element from a list in Dart, I can confirm this this getRandomListElement
method works:
As a quick note, here are a couple of examples of how to simulate a slow-responding Flutter/Dart method:
Future<bool> _getFutureBool() {
return Future.delayed(Duration(milliseconds: 500))
.then((onValue) => true);
}
Future<bool> getEnableNotifications() async {
final SharedPreferences prefs = await SharedPreferences.getInstance();
return Future.delayed(const Duration(milliseconds: 1000), () {
return prefs.getBool(KEY_ENABLE_NOTIFICATIONS) ?? false;
});
}
As shown, the first example uses Future.delayed
followed by a then
call, and the second example shows Future.delayed
with a lambda function.
You’ll want to use this code/technique when you’re working with Flutter futures and its FutureBuilder
class.
I was reminded about the need to test things likes futures and FutureBuilder
when some code I found on the internet wasn’t working properly. As a result I wrote my tutorial, How to correctly create a Flutter FutureBuilder.
As a quick note, in the Dart programming language, the ternary operator syntax is the same as the Java ternary operator syntax. The general syntax is:
result = testCondition ? trueValue : falseValue
Dart ternary operator syntax examples
A few examples helps to demonstrate Dart’s ternary syntax:
“All things that appear in this world are illusion. If you view all appearance as nonappearance, you will see your true nature.”
~ From the Diamond Sutra, via
Wanting Enlightenment is a Big Mistake (#ad)
“There he goes. One of God's own prototypes. A high-powered mutant of some kind never even considered for mass production. Too weird to live, and too rare to die.”
~ Hunter S. Thompson, Fear and Loathing in Las Vegas (#ad)
If you’re interested in Dart/Flutter programming, here’s a little example of how to pass a Dart function into another function or method. The solution is basically a three-step process.
Step 1: Define a Dart method that takes a function parameter
First, define a Dart method that takes a function as a parameter, such as this exec
method: