
Learn Scala 3,
Functional Programming,
and ZIO 2.
Free video training courses by Alvin Alexander,
creator of books on Scala and Functional Programming.
Sponsored by:
Alvin’s Courses
Click an image below to visit the “Courses” page.
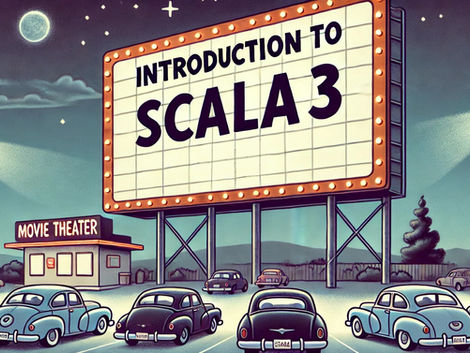
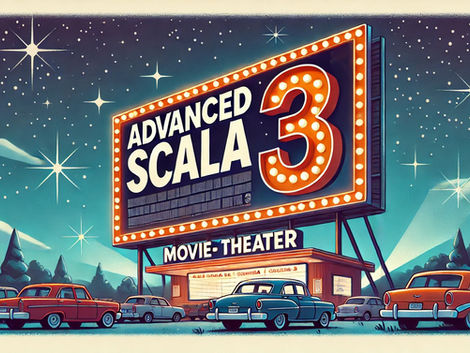

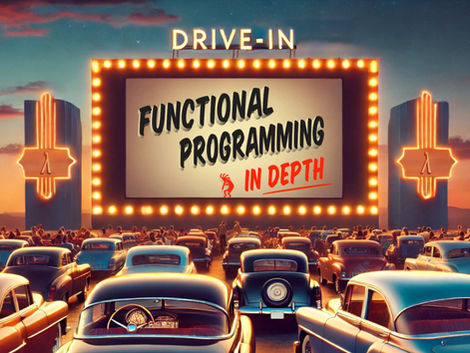
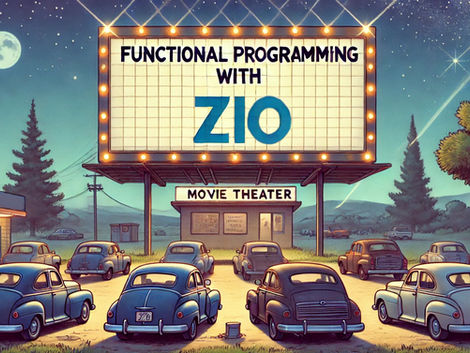
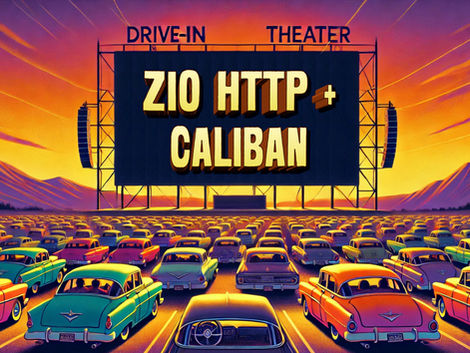
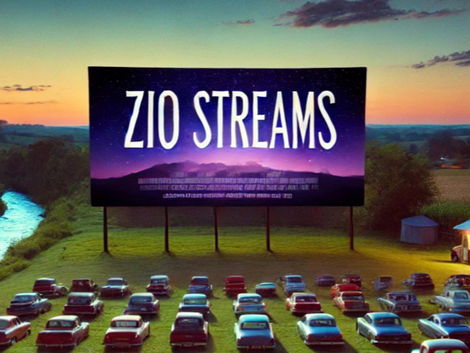
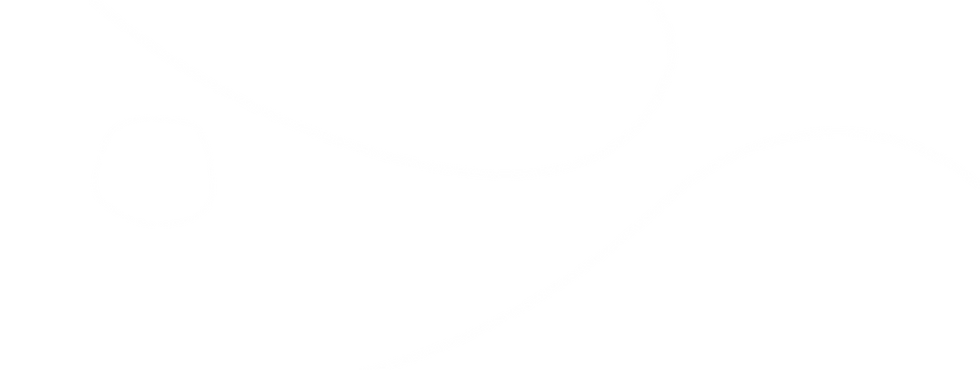
The Impact of Learning Scala
Learning Scala 3, Functional Programming, and ZIO 2 can provide huge benefits to you. Functional programming techniques can help you write code that’s easier to write, read, test, and maintain, and Scala’s fusion of OOP and FP will change the way you think about the code you write.
Enhances Problem-Solving Skills
Learning Scala enhances analytical thinking and problem-solving abilities. Scala helps you to “think different”, and broaden your problem-solving toolkit.
Opens Career Opportunities
Scala proficiency opens doors to fun, high-paying, diverse career opportunities in software development and engineering.
Versatility in Approach
When you know both FP and OOP, you become more versatile, and learn when to use each paradigm.
Fosters Innovation
Since its inception, Scala has been at the forefront of cutting-edge programming technologies, ahead of other languages by many years.
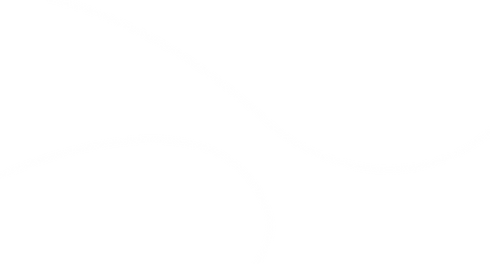
“Scala encourages you to think differently. It lets you focus on solving problems, rather than wrestling with the language itself.”
~ David Pollak,
author, Beginning Scala

“Scala lets you grow into your own capabilities, empowering you to write highly scalable and maintainable software.”
~ Martin Odersky,
Creator of the Scala Language