With custom icon
It’s important to note that you can also customize the icon that is displayed when using a JOptionPane
showMessageDialog
. In the following example, I specify that I want to display my own PNG image as an icon on the dialog by creating an ImageIcon
as an additional argument to the showMessageDialog
method:
import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JOptionPane; public class ShowMessageDialogExample1 { public static void main(String[] args) { // create our jframe JFrame frame = new JFrame("JOptionPane showMessageDialog example"); // show a joptionpane dialog using showMessageDialog JOptionPane.showMessageDialog(frame, "Your RSS feed has been published", "RSS Feed Published", JOptionPane.INFORMATION_MESSAGE, new ImageIcon(ShowMessageDialogExample1.class.getResource("Feeds_Green_32x32.png"))); System.exit(0); } }
This results in the following dialog:
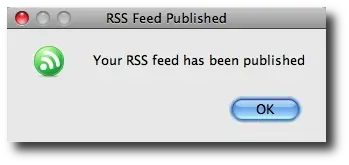
(As a brief aside, it always amazes me how much different an icon makes to a dialog. Write text all you want, but one change to an icon produces extremely different feelings for text that is otherwise the same.)
Real world code: JOptionPane in addActionListener
In a more real-world use of the JOptionPane
showMessageDialog
method, you’ll typically display a message dialog when a GUI event is triggered. In Java, you’ll typically add an ActionListener
to a GUI component (like a JButton
), and then define your message dialog within the actionPerformed
method of that ActionListener
. An example of this is shown in the following JOptionPane
example:
printButton.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent event) { JOptionPane.showMessageDialog((Component)event.getSource(), "Your message goes here", "Title goes here", JOptionPane.INFORMATION_MESSAGE); } });
This is pretty typical of how a JOptionPane
is shown when a GUI event is triggered.
Three showMessageDialog
method signatures
For your reference, it may help to know that as of Java 6, the showMessageDialog
has three different method signatures. The first showMessageDialog
syntax looks like this:
showMessageDialog(Component parentComponent, Object message)
The second JOptionPane
showMessageDialog
syntax looks like this:
showMessageDialog(Component parentComponent, Object message, String title, int messageType)
And the third JOptionPane
showMessageDialog
signature looks like this:
showMessageDialog(Component parentComponent, Object message, String title, int messageType, Icon icon)
You can add components as well
As a final (and very important) note, you may have noticed that those JOptionPane
showMessageDialog
signatures include this subtle component:
Object message
Many people don’t know it, but your message
can be many different things, they don’t have to be just messages of the Java String
type. You can take advantage of this by showing Java components in your message dialogs, and I’ll demonstrate that in future JOptionPane
showMessageDialog
examples.